3D.Compute.Generate.Torus
This node generates a torus with a fixed outer radius of 1.0. The tube radius slot allows selecting the inner radius in percentage of the outer radius.
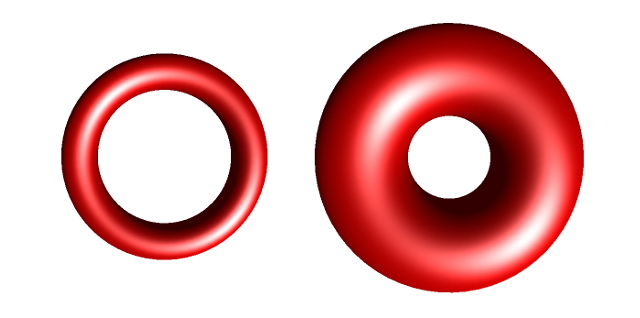
The image above shows two tori with tube radius 20% (left) and tube radius 50% (right).
3D.Compute.Generate.TorusKnot
This node generates a torus knot. The knot is constructed from a 3D curve that twines around an imagined torus. The imagined torus has a fixed outer radius of 1.0 and its inner radius can be selected via the torus radius slot in percentage of the outer radius. The twinded 3D curve is thickened in order to form a tube. The radius of the tube's cross sections can be selected with the tube radius slot in percentage of the outer radius.
How often the 3D curve twines around the imagined torus is controlled by two integers values p and q. The parameter p can be selected with the winding up/down slot and q with the winding around slot. Some (p-q)-torus knots have special names, e.g. a (2,3)-torus knot is called trefoil knot and a (2,5)-torus knot is called cinquefoil knot.
![]() (1,0)-torus knot |
![]() (1,5)-torus knot |
![]() (1,7)-torus knot |
![]() (1,9)-torus knot |
![]() (1,11)-torus knot |
![]() (2,3)-torus knot |
![]() (2,5)-torus knot |
![]() (2,7)-torus knot |
![]() (2,9)-torus knot |
![]() (2,11)-torus knot |
![]() (3,4)-torus knot |
![]() (3,5)-torus knot |
![]() (3,7)-torus knot |
![]() (3,8)-torus knot |
![]() (3,11)-torus knot |
What follows below is some math that I wrote down while implementing these nodes:
Torus
A torus can be constructed from a 2D parameterization in $\theta$ and $\phi$, both in range $[0, 2\pi]$. The variable $\theta$ follows the outer circle, and $\phi$ the inner (cross-section) circles as shown in the image below.
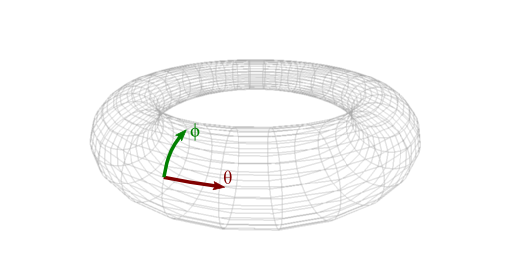
With this parameterization the 3D vertices of a torus are computed by: